PROGRAM #3
Fall 2010
Due: October 29, 2010, 5pm on Angel
General Guidelines:
- All source code must be in
C++. You can use Windows or Unix environments at
your discretion.
- Each program project should
be accompanied by a COVER SHEET (see details below). Assignments without
cover page will NOT be graded.
- This is an individual
programming assignment. No team work is allowed.
- The final material for
submission should be entirely written by you. If you decide to consult
with others or refer materials online, you MUST give due credits to these
sources (people, books, webpages, etc.) by
listing them on the cover sheet mentioned below. Note that no points will
be deducted for referencing these sources. However, your
discussion/consultation should be limited to the initial design level.
Sharing or even showing your source code/assignment verbiage to anyone
else in the class, or direct reproduction of source code/verbiage from
online resources, will all be considered plagiarism, and therefore will be
awarded ZERO points and subject to the WSU Academic Dishonesty policy.
(Reproducing from the Weiss textbook is an exception to this rule, and
such reproduction is encouraged wherever possible.)
- Grading will be based on
correctness, coding style, implementation efficiency, exception handling,
source code documentation, and the written report.
- Angel Submission:
All assignments with cover sheet should be zipped or tarred into one
archive folder (named after your last name), and attached by email and
sent to:
"All course faculty"
through Angel. This will send out an email to both the
instructor and the TA. Submissions are due by 5pm.
PS: Submissions through any other means or from general
email addresses will be discarded and will NOT be graded.
So please follow the above instructions carefully.
Late submission policy: A late penalty of 10% will be assessed for
late submissions within the next 24-hour. Note: Submissions will be
accepted only through the Angel webmail. Any other submission outside the
Angel portal will be discarded and not graded. (In the unlikely event of
an Angel server outage on the day of submission, assisgnments
can be emailed to the instructor's EECS email account.)
-
PROBLEM A board game
For this assignment, you will be implementing a simple board game, as per the
specifications stated below, using the best data structure of your choice.
Specifications:
A "board" is defined as a m * m square matrix, with each cell
identified using a unique (x,y) coordinate. An example 10
* 10 board is shown in the figure below. In a game, a board has n players, where n ≤ m always. The
figure shown below is for m=10 and n=8.
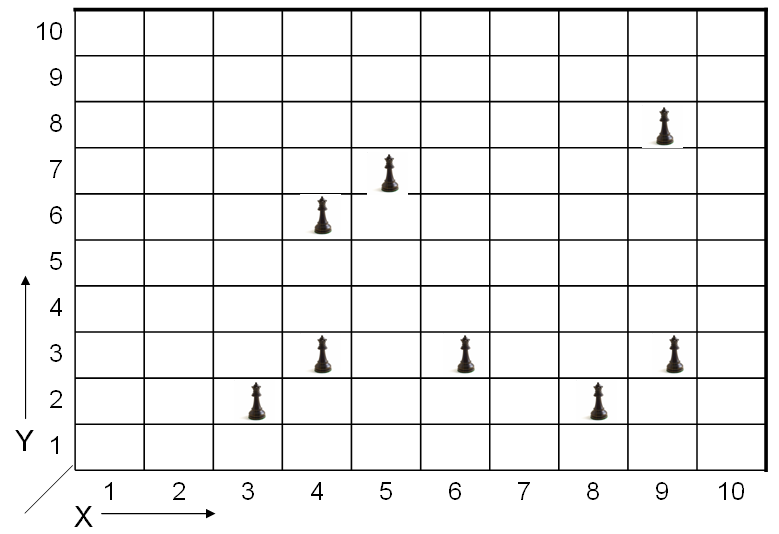
Your task is to implement the following specifications and functionalities:
- Write a Board class
that implements a board of size m *
m with n players. The value of
m is user specified (during class construction). At the start of the game,
all the m * m cells of the board
are empty (i.e., n=0). As the
game progresses n is allowed to
change.
- Write a Player class
that implements each player. Each player object will have a unique,
positive integer ID, and at any given time the player will occupy a
unique (x,y) position on the board. Care must be
taken that the value of (x,y) position is always
within bounds of the board at all times. Also, no two players are allowed to share the same cell.
- Implement an Insert
method in the Board class that will allow you to add a new player to the
board. The method should take as input the player ID to be inserted, along
with a desired (x,y) position into which it is to be
initially placed. For successful insertion, i) a
player with the same ID should not
already exist on the board; AND ii)
the specified insertion position on board should be currently
unoccupied. A third condition would be to ensure the total number of players
with this insertion (i.e., n) should not exceed m. If successful, the method should update n and return
true. If insertion fails, the
code should display an appropriate error message and return false without changing anything.
- Implement a Remove method
in the Board class that will allow you to remove a player from the board.
The method should take as input the player ID to be removed, and should
return true upon successful
removal and false otherwise
(i.e., player not found). The value of n
should also be accordingly updated. Note that upon successful
removal, the corresponding cell on the board should be released to being
unoccupied.
- Implement a Find method
in the Board class that is given a player ID and returns true if the player is found and false otherwise.
- Implement a MoveTo method in the Board class that
takes as input a player ID and a destination (x,y) cell
position. The method should first locate the player, and move the player
from its current position, say (x1,y1),
to the new position (x,y) only if:
- (x,y) is within bounds, and
- the
movement from (x1,y1) to (x,y) is always along
the same row or same column or same diagonal in any direction (i.e.,
top-left -- right-bottom or top-right -- left-bottom).
Additional action is necessary if the destination cell (x,y)
already has some other player, say Y. Then this function should first remove Y
from the board before placing this player (ID) in its new position. Upon a
successful move, the method should return true.
If any player was removed in the process, the method should print a message to
indicate which player was removed. If the move fails, the code should display
an error message stating the reason of failure and return false.
Note: Any player(s) on the board along the path of moving
from (x1,y1) to (x,y) is/are
left unaffected by this move.
- Implement a PrintByCoordinate method in the Board class
that prints all the player IDs along with their (x,y)
positions, in the increasing order of their x-coordinate values. If there are many players with the same x-coordinate value then you may
print them in any order. In the example illustrated in the figure above,
this method can print the player IDs from the positions (in this
order): (3,2) (4,3) (4,6) (5,7) (6,3) (8,2) (9,3) (9,8).
Another equally correct output (among other possibilities) is: (3,2)
(4,6) (4,3) (5,7) (6,3) (8,2) (9,3) (9,8).
Note: The print function should display only occupied positions. Do
not print the entire m * m
matrix.
- Implement a PrintByID method in the Board class that prints
all the player IDs along with their (x,y) positions, in the
increasing order of their IDs. Again, the print should not display any unoccupied
positions.
- Write a main function
in which you test all the above methods at least once. You can think of
using the example in the figure above as an initial configuration to do
testing. (We will have our own test code to test your source. Test sample here) There will be only
correctness/functionality testing in this exercise. No need to print any
timing stats, although performance will be assessed at the design level.
Design:
It is expected that you use balanced binary
search trees for this project. However, you are free to pick the STL container
or containers as appropriate (set, map, multiset,
multimap). But your choice should be based on what is
expected to give the best performance (both time and memory) in practice. And
your design is required to take the following assumptions into consideration:
i)
In practice, you can expect n to be much smaller than m.
(For example, n=10, m=1,000,000). This
simply implies that your code should not
store the whole m*m board because it could run out of memory.
ii)
You can assume that the number of players sharing the
same x-value is going to be a small constant.
You are permitted to maintain multiple data structures and/or copies of the
same data in different containers as you deem necessary for design and
efficiency. You are free to define new member functions not specified above to
enhance code reuse and modularity.
Written Report:
In a separate document (Word or PDF), compile the following sections:
·
A: Problem
statement. In a couple of sentences state the goal(s) of this exercise.
- B: Experimental setup. In this section, specify the machine
architectural details where the testing was conducted. Also mention
whether you used Windows or Unix or Mac OSX for
your testing.
·
C:Algorithm
design (limit to 2 pages): Provide a design document for your code with the
following information. State what data container(s) you used to support the
different functions and why; what is the run-time complexity of each of the
functions in your code; and what is the memory complexity for your
implementation as a whole. Figures and illustrations can be used to show the
design.
FINAL CHECKLIST FOR SUBMISSION:
___ Cover sheet
___ A separate folder
containing all your sourcecode (including the main
function you used for testing)
___ Report
___ Both the above zipped
into another folder archivecalled Program3<YourLastNames>.zip