-
Create the following two functions:
sumN(n) returns the sum of the first n natural numbers.
sumNCubes(n) returns the sum of the cubes of the first n natural numbers.
Then use these functions in a program that prompts a user for n and prints out the sum of the first n natural numbers and the sum of the cubes of the first n natural numbers. Your functions should do something reasonable if the user enters a floating point number (e.g., 2.5) or a negative number (e.g., -2).
-
Create the following two functions:
sphereArea(radius) returns the surface of a sphere having the given radius.
sphereVolume(radius) returns the volume of a sphere having the given radius.
Ask the user for a radius and use your funcitons to calculate the volume and surface area of a sphere. You can assume the user does not enter a negative value for the radius.
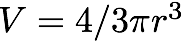
-
Create the following function:
toNumbers(strList)
This function takes strList as a parameter, which is a list of strings. Each string represents a number. The function should modify each entry in the list by converting it to a number and return the modified list. Be sure to test all possible cases. If there are some inputs that your code does not correctly handle, note this in the comments. Your main function should call this function a number of times with different inputs to show that your function works correctly.